목차
-
클래스 & 생성자 (Classes & Constructors)
-
1) prototype 을 직접 조작하는것을 피하고 항상 class 를 사용하세요.
-
2) 상속에는 extends를 사용하세요.
-
3) 메소드가 this를 반환하게 함으로써 메소드 체이닝을 할 수 있습니다.
-
4) toString()을 사용해도 되지만, 올바르게 동작하는지와 사이드 이펙트가 없는지 확인해 주세요.
-
5) 클래스는 생성자가 명시되지 않은 경우 기본 생성자를 갖습니다. 빈 생성자 함수나 부모 클래스로 위임하는 함수는 불필요합니다.
-
-
6) 중복되는 클래스 멤버를 만들지 마세요
반응형
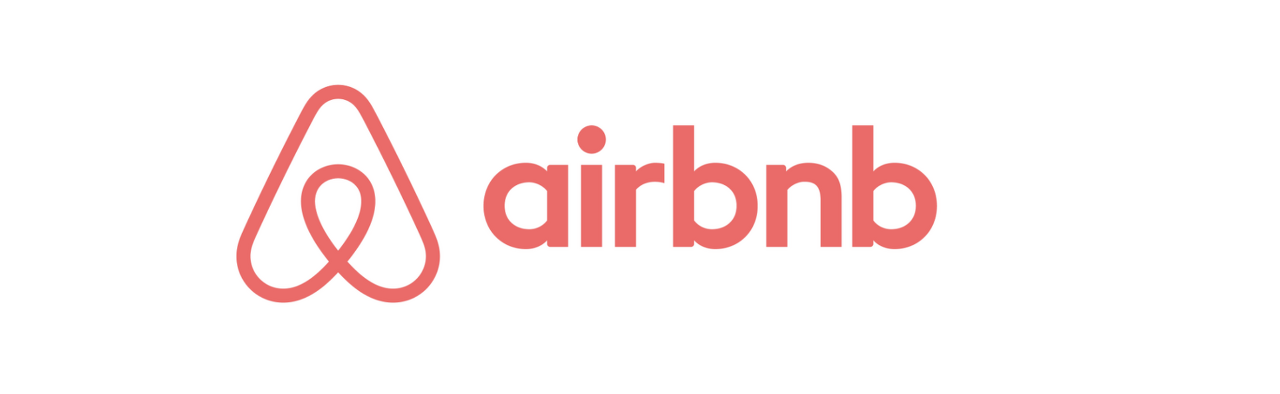
우아한테크코스의 자바스크립트 스타일 가이드는 Airbnb 자바스크립트 스타일 가이드를 기준으로 하기에
Airbnb 자바스크립트 스타일 가이드 기준을 보고 이해를 바탕으로 필사하였으며 경중에따라 가감하였습니다.
클래스 & 생성자 (Classes & Constructors)
1) prototype 을 직접 조작하는것을 피하고 항상 class 를 사용하세요.
왜? class 구문은 간결하고 의미를 알기 쉽기 때문입니다.
// bad
function Queue(contents = []) {
this.queue = [...contents];
}
Queue.prototype.pop = function () {
const value = this.queue[0];
this.queue.splice(0, 1);
return value;
};
// good
class Queue {
constructor(contents = []) {
this.queue = [...contents];
}
pop() {
const value = this.queue[0];
this.queue.splice(0, 1);
return value;
}
}
2) 상속에는 extends를 사용하세요.
왜? instanceof 를 파괴하지 않고 프로토타입 상속을 하기 위해 내장된 방법이기 때문입니다.
// bad
const inherits = require('inherits');
function PeekableQueue(contents) {
Queue.apply(this, contents);
}
inherits(PeekableQueue, Queue);
PeekableQueue.prototype.peek = function () {
return this.queue[0];
};
// good
class PeekableQueue extends Queue {
peek() {
return this.queue[0];
}
}
3) 메소드가 this를 반환하게 함으로써 메소드 체이닝을 할 수 있습니다.
// bad
Jedi.prototype.jump = function () {
this.jumping = true;
return true;
};
Jedi.prototype.setHeight = function (height) {
this.height = height;
};
const luke = new Jedi();
luke.jump(); // => true
luke.setHeight(20); // => undefined
// good
class Jedi {
jump() {
this.jumping = true;
return this;
}
setHeight(height) {
this.height = height;
return this;
}
}
const luke = new Jedi();
luke.jump()
.setHeight(20);
4) toString()을 사용해도 되지만, 올바르게 동작하는지와 사이드 이펙트가 없는지 확인해 주세요.
class Jedi {
constructor(options = {}) {
this.name = options.name || 'no name';
}
getName() {
return this.name;
}
toString() {
return `Jedi - ${this.getName()}`;
}
}
5) 클래스는 생성자가 명시되지 않은 경우 기본 생성자를 갖습니다. 빈 생성자 함수나 부모 클래스로 위임하는 함수는 불필요합니다.
// bad
class Jedi {
constructor() {}
getName() {
return this.name;
}
}
// bad
class Rey extends Jedi {
constructor(...args) {
super(...args);
}
}
// good
class Rey extends Jedi {
constructor(...args) {
super(...args);
this.name = 'Rey';
}
}
6) 중복되는 클래스 멤버를 만들지 마세요
// bad
class Foo {
bar() { return 1; }
bar() { return 2; }
}
// good
class Foo {
bar() { return 1; }
}
// good
class Foo {
bar() { return 2; }
}
7) 클래스 메소드는 외부 라이브러리나 프레임워크가 구체적으로 비정적 메소드를 요구하지 않는 이상 this를 사용하거나 해당 메소드를 정적 메소드로 만들어야 합니다. 인스턴스 메서드는 수신자의 속성에 따라 다르게 동작함을 나타내야 합니다.
// bad
class Foo {
bar() {
console.log('bar');
}
}
// good - this를 사용했습니다
class Foo {
bar() {
console.log(this.bar);
}
}
// good - constructor가 면제됩니다
class Foo {
constructor() {
// ...
}
}
// good - 정적 메소드는 this를 사용하지 않는다고 예상할 수 있습니다
class Foo {
static bar() {
console.log('bar');
}
}
반응형
'정보 > Airbnb JS Code Style Guide' 카테고리의 다른 글
[Airbnb JS Style Guide] 모듈 (Modules) (0) | 2022.11.06 |
---|---|
[Airbnb JS Style Guide] 객체 , Types, References (0) | 2022.11.03 |
[Airbnb JS Style Guide] 주석을 사용해야한다면 어떻게 사용해야할까? (0) | 2022.11.03 |
[Airbnb JS Style Guide] 당신의 함수는 안녕하십니까? (0) | 2022.11.03 |
반응형
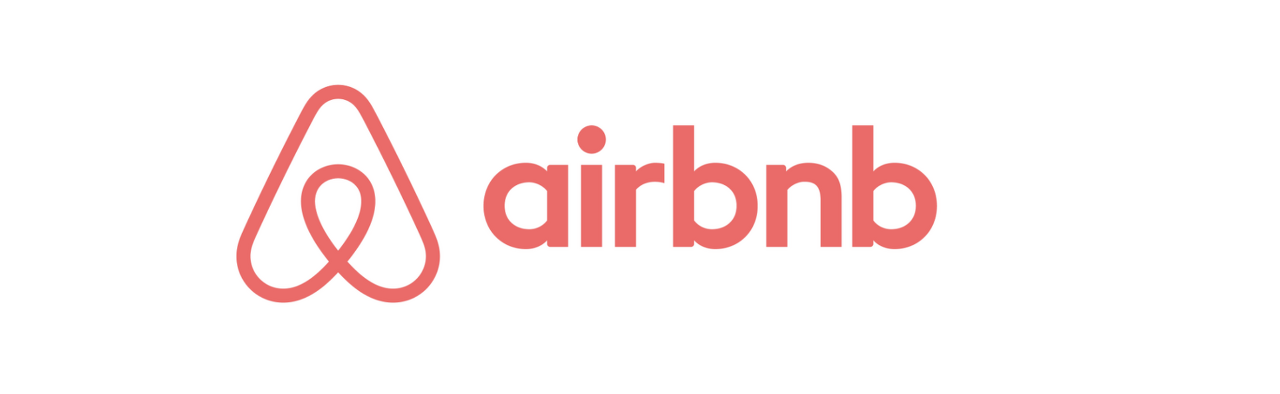
우아한테크코스의 자바스크립트 스타일 가이드는 Airbnb 자바스크립트 스타일 가이드를 기준으로 하기에
Airbnb 자바스크립트 스타일 가이드 기준을 보고 이해를 바탕으로 필사하였으며 경중에따라 가감하였습니다.
클래스 & 생성자 (Classes & Constructors)
1) prototype 을 직접 조작하는것을 피하고 항상 class 를 사용하세요.
왜? class 구문은 간결하고 의미를 알기 쉽기 때문입니다.
// bad function Queue(contents = []) { this.queue = [...contents]; } Queue.prototype.pop = function () { const value = this.queue[0]; this.queue.splice(0, 1); return value; }; // good class Queue { constructor(contents = []) { this.queue = [...contents]; } pop() { const value = this.queue[0]; this.queue.splice(0, 1); return value; } }
2) 상속에는 extends를 사용하세요.
왜? instanceof 를 파괴하지 않고 프로토타입 상속을 하기 위해 내장된 방법이기 때문입니다.
// bad const inherits = require('inherits'); function PeekableQueue(contents) { Queue.apply(this, contents); } inherits(PeekableQueue, Queue); PeekableQueue.prototype.peek = function () { return this.queue[0]; }; // good class PeekableQueue extends Queue { peek() { return this.queue[0]; } }
3) 메소드가 this를 반환하게 함으로써 메소드 체이닝을 할 수 있습니다.
// bad Jedi.prototype.jump = function () { this.jumping = true; return true; }; Jedi.prototype.setHeight = function (height) { this.height = height; }; const luke = new Jedi(); luke.jump(); // => true luke.setHeight(20); // => undefined // good class Jedi { jump() { this.jumping = true; return this; } setHeight(height) { this.height = height; return this; } } const luke = new Jedi(); luke.jump() .setHeight(20);
4) toString()을 사용해도 되지만, 올바르게 동작하는지와 사이드 이펙트가 없는지 확인해 주세요.
class Jedi { constructor(options = {}) { this.name = options.name || 'no name'; } getName() { return this.name; } toString() { return `Jedi - ${this.getName()}`; } }
5) 클래스는 생성자가 명시되지 않은 경우 기본 생성자를 갖습니다. 빈 생성자 함수나 부모 클래스로 위임하는 함수는 불필요합니다.
// bad class Jedi { constructor() {} getName() { return this.name; } } // bad class Rey extends Jedi { constructor(...args) { super(...args); } } // good class Rey extends Jedi { constructor(...args) { super(...args); this.name = 'Rey'; } }
6) 중복되는 클래스 멤버를 만들지 마세요
// bad class Foo { bar() { return 1; } bar() { return 2; } } // good class Foo { bar() { return 1; } } // good class Foo { bar() { return 2; } }
7) 클래스 메소드는 외부 라이브러리나 프레임워크가 구체적으로 비정적 메소드를 요구하지 않는 이상 this를 사용하거나 해당 메소드를 정적 메소드로 만들어야 합니다. 인스턴스 메서드는 수신자의 속성에 따라 다르게 동작함을 나타내야 합니다.
// bad class Foo { bar() { console.log('bar'); } } // good - this를 사용했습니다 class Foo { bar() { console.log(this.bar); } } // good - constructor가 면제됩니다 class Foo { constructor() { // ... } } // good - 정적 메소드는 this를 사용하지 않는다고 예상할 수 있습니다 class Foo { static bar() { console.log('bar'); } }
반응형
'정보 > Airbnb JS Code Style Guide' 카테고리의 다른 글
[Airbnb JS Style Guide] 모듈 (Modules) (0) | 2022.11.06 |
---|---|
[Airbnb JS Style Guide] 객체 , Types, References (0) | 2022.11.03 |
[Airbnb JS Style Guide] 주석을 사용해야한다면 어떻게 사용해야할까? (0) | 2022.11.03 |
[Airbnb JS Style Guide] 당신의 함수는 안녕하십니까? (0) | 2022.11.03 |